Tables
Tables are used to group informations in various rows and columns together. The basic table looks like this::
<table> <thead> <tr> <th>Age</th> <th>Salary</th> </tr> </thead> <tbody> <tr> <td>Row 1, Column 1</td> <td>Row 1, Column 2</td> </tr> <tr> <td>Row 2, Column 1</td> <td>Row 2, Column 2</td> </tr> </tbody> <tfoot> <tr> <th>Total</th> <th>345</th> </tr> </tfoot> </table>
It looks so complex, Is not it ?
<table> tag has 3 childs : thead, tbody and tfoot, where tfoot
is rarely used so in practice, we use only thead and tbody.
So now it looks something like this ::
<table> <thead> ...... </thead> <tbody> ...... </tbody> </table>
As thead represents the header of the table, it will wrap only one row (tr) inside it.
And again this tr will wrap as many th as it needs column header.
th means header cells and it exists in thead.
In terms of code, kindly have a look below::
<table> <thead> <tr> <th>Age</th> <th>Salary</th> </tr> </thead> </table>
tbody represents the body of the table, it will wrap as many rows as it needs (tr) inside it.
And again this tr will wrap as many td as it needs column headers data.
td means data cells and it exists in tbody.
In terms of code, kindly have a look below::
<table> <tbody> <tr> <td>Row 1, Column 1</td> <td>Row 1, Column 2</td> </tr> <tr> <td>Row 2, Column 1</td> <td>Row 2, Column 2</td> </tr> </tbody> </table>
Now we understood the tables and how to write it. But we need to understand some specific attributes on table tag and what is the purpose of it.
- border attribute and border-collapse styling to collapse double borders is the firsr and very important concept in html table.
- colspan attribute : If this attribute is used on a th or td cells then
those cells will merge those columns as one column.
e.g : colspan = "4" will merge 4 cells(th/td) into one cell. And this property always used on th/td only. - rowspan attribute : If this attribute is used on a th or td cells then
those cells will merge those rows as one row.
e.g : rowspan = "4" will merge 4 cells(th/td) into one cell. And this property always used on td only. - cellpadding attribute : This attribute is applied on a table tag and it will give padding to all the th/th cells.
- cellspacing attribute : This attribute is applied on a table tag and it will give spaces/margin between different cells.
Now below we will see examples of all of the above attributes in action::
Example of border attribute and border-collapse styling
<table border="1" style="border-collapse: collapse"> <thead> <tr> <th>Column 1</th> <th>Column 2</th> <th>Column 3</th> </tr> </thead> <tbody> <tr> <td>Row 1 Cell 1</td> <td>Row 1 Cell 2</td> <td>Row 1 Cell 3</td> </tr> <tr> <td>Row 2 Cell 1</td> <td>Row 2 Cell 2</td> <td>Row 2 Cell 3</td> </tr> <tr> <td>Row 3 Cell 1</td> <td>Row 3 Cell 2</td> <td>Row 3 Cell 3</td> </tr> </tbody> </table>
Now focus that on table tag, we have border="1", meaning border of table is set to 1px,
and then you see a double border. Once you apply a styling of border-collapse: collapse
then double borders are collapsed.
Note :
Here we are using html style attribute to do styling, but in practice we write
this style in a css file.
I am attaching 2 images here , one for double border and then collapse of double borders, which is what
we want.
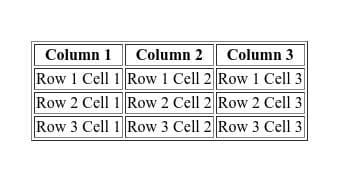
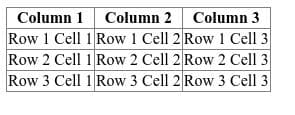
Example of colspan and rowspan attribute
We can use colspan and rowspan attribute separately also, based on businedd requirement or figma design. But here i will show you both used together and explain you in detail.
<table border="1" style="border-collapse: collapse"> <thead> <tr> <th>col 1</th> <th>col 2</th> <th>col 3</th> </tr> </thead> <tbody> <tr> <td rowspan = "2">Row 1 Cell 1</td> <td>row 1 Cell 2</td> <td>row 1 Cell 3</td> </tr> <tr> <td>row 2 Cell 2</td> <td>row 2 Cell 3</td> </tr> <tr> <td colspan = "3">row 3 Cell 1</td> </tr> </tbody> </table>
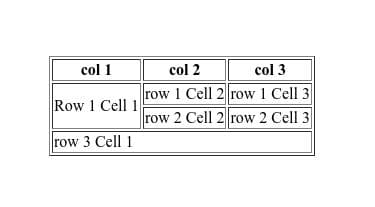
As it is very simple to understand, here in the 3rd row(tr), we have only one td, but in thead, the maximum
columns we have is 3, so we are trying to merge 3 columns cells into one with colspan = "3" attribute.
Now as for rowspan usage, in the first row in tbody the attribute rowspan = "2", will merge 2
row cells into one:: row 1 cell 1 and row 2 cell 1 are merged into one cell row 1 cell 1.